Why Should We Prevent A Clientã¢â‚¬â„¢s Direct Access To A Data Structure?
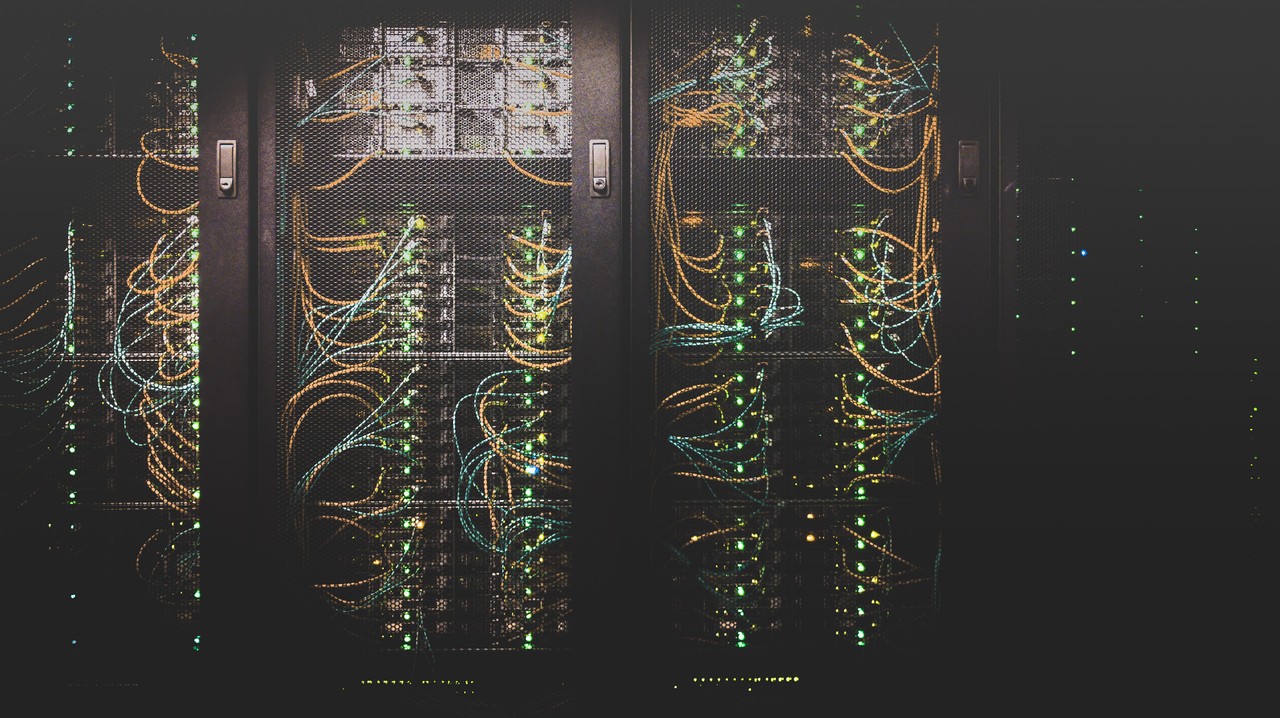
Ever wondered how login/signup on a website works on the back-finish? Or how when you search for "beautiful kitties" on YouTube, you get a bunch of results and are able to stream off of a remote machine?
In this beginner friendly guide, I will walk you through the process of setting upward a RESTful API. We'll declassify some of the jargon and take a look at how we can code a server in NodeJS. Let'due south swoop a bit deeper into JavaScript!
Get that jargon abroad
Then, what is REST? Co-ordinate to Wikipedia:
Representational state transfer (Balance) is a software architectural style that defines a set of constraints to exist used for creating Web services. RESTful Spider web services allow the requesting systems to admission and manipulate textual representations of Spider web resource by using a uniform and predefined set up of stateless operations
Let's demystify what that ways (hopefully you got the full form). REST is basically a prepare of rules for communication between a client and server. In that location are a few constraints on the definition of Rest:
- Customer-Server Architecture: the user interface of the website/app should be separated from the data request/storage, and then each role can be scaled individually.
- Statelessness: the communication should accept no client context stored on server. This ways each request to the server should be made with all the required information and no assumptions should be made if the server has any data from previous requests.
- Layered system: client should not be able to tell if it is communicating directly with the server or some intermediary. These intermediary servers (be it proxy or load balancers) allow for scalability and security of the underlying server.
Okay, then now that you know what RESTful services are, hither are some of the terms used in the heading:
- Rest Client: code or an app that can access these REST services. You are using one right now! Yes, the browser can act as an uncontrolled Balance customer (the website handles the browser requests). The browser, for a long time, used an in-built role called XMLHttpRequest for all REST requests. Merely, this was succeeded by FetchAPI, a modern, promise based approach to requests. Others examples are code libraries like axios, superagent and got or some dedicated apps similar Postman (or an online version, postwoman!), or a control line tool like cURL!.
- Residual Service: the server. At that place are many pop libraries that brand cosmos of these servers a breeze, similar ExpressJS for NodeJS and Django for Python.
- REST API: this defines the endpoint and methods immune to access/submit information to the server. We will talk about this in great particular below. Other alternatives to this are: GraphQL, JSON-Pure and oData.
So tell me now, how does REST expect?
In very wide terms, you ask the server for a sure data or ask it to save some data, and the server responds to the requests.
In programming terms, there is an endpoint (a URL) that the server is waiting to become a request. We connect to that endpoint and ship in some information nearly us (remember, Remainder is stateless, no data about the asking is stored) and the server responds with the correct response.
Words are tiresome, let me requite yous a demonstration. I will exist using Postman to show you the request and response:

The returned data is in JSON (JavaScript Object Notation) and tin be accessed directly.
Hither, https://official-joke-api.appspot.com/random_joke
is called an endpoint of an API. There volition exist a server listening on that endpoint for requests like the 1 we made.
Beefcake of REST:
Alright, so now we know that information can exist requested by the client and the server will respond appropriately. Let'south look deeper into how a request is formed.
- Endpoint: I take already told you about this. For a refresher, information technology is the URL where the REST Server is listening.
- Method: Earlier, I wrote that y'all can either asking data or modify it, but how will the server know what kind of operation the client wants to perform? REST implements multiple 'methods' for dissimilar types of request, the post-obit are nigh popular:
- Become: Get resource from the server.
- POST: Create resources to the server.
- PATCH or PUT: Update existing resources on the server.
- DELETE: Delete existing resource from the server.
- Headers: The additional details provided for communication between client and server (remember, REST is stateless). Some of the common headers are:
Request:
- host: the IP of customer (or from where request originated)
- accept-language: linguistic communication understandable by the client
- user-agent: data almost customer, operating system and vendor
Response:
- status: the condition of request or HTTP code.
- content-blazon: blazon of resource sent by server.
- set-cookie: sets cookies by server - Information: (also called body or bulletin) contains info y'all want to transport to the server.
Plenty with the details – show me the code.
Permit's begin coding a REST Service in Node. We will be implementing all the things we learnt above. We will also be using ES6+ to write our service in.
Brand sure you have Node.JS installed and node
and npm
are available in your path. I will exist using Node 12.16.ii and NPM half dozen.14.iv.
Create a directory remainder-service-node
and cd into information technology:
mkdir rest-service-node cd rest-service-node
Initialize the node project:
npm init -y
The -y
flag skips all the questions. If you lot desire to fill in the whole questionnaire, only run npm init
.
Let'due south install some packages. We volition be using the ExpressJS framework for developing the REST Server. Run the post-obit command to install information technology:
npm install --save express body-parser
What's torso-parser
at that place for? Limited, by default, is incapable of handling data sent via POST asking every bit JSON. body-parser
allows Limited to overcome this.
Create a file called server.js
and add the following code:
const express = require("express"); const bodyParser = require("body-parser"); const app = express(); app.apply(bodyParser.json()); app.heed(5000, () => { panel.log(`Server is running on port 5000.`); });
The kickoff two lines are importing Express and body-parser.
Third line initializes the Express server and sets it to a variable called app
.
The line, app.use(bodyParser.json());
initializes the body-parser plugin.
Finally, nosotros are setting our server to listen on port 5000
for requests.
Getting information from the REST Server:
To get information from a server, nosotros demand a Get
request. Add the following code before app.listen
:
const sayHi = (req, res) => { res.send("Howdy!"); }; app.go("/", sayHi);
We have created a office sayHi
which takes ii parameters req
and res
(I volition explain later on) and sends a 'Hi!' as response.
app.become()
takes 2 parameters, the road path and function to telephone call when the path is requested past the customer. So, the last line translates to: Hey server, listen for requests on the '/' (think homepage) and call the sayHi
function if a request is made.
app.get
also gives the states a asking
object containing all the data sent past the client and a response
object which contains all the methods with which nosotros can respond to the client. Though these are accessible as function parameters, the general naming convention suggests nosotros name them res
for response
and req
for asking
.
Plenty chatter. Let'due south fire up the server! Run the following server:
node server.js
If everything is successful, you should see a bulletin on console saying: Server is running on port 5000.
Note: You lot can alter the port to whatever number you want.

Open up your browser and navigate to http://localhost:5000/
and you should encounter something like this:

At that place you become! Your get-go Become
request was successful!
Sending data to REST Server:
As we have discussed earlier, allow'due south setup how we can implement a POST
request into our server. We volition be sending in ii numbers and the server will render the sum of the numbers. Add together this new method below the app.become
:
app.post("/add", (req, res) => { const { a, b } = req.body; res.transport(`The sum is: ${a + b}`); });
Here, nosotros volition exist sending the information in JSON format, like this:
{ "a":5, "b":10 }
Let'south go over the code:
On line 1, we are invoking the .mail()
method of ExpressJS, which allows the server to listen for Post
requests. This function takes in the same parameters as the .go()
method. The route that nosotros are passing is /add
, and so i can access the endpoint as http://your-ip-accost:port/add together
or in our case localhost:5000/add
. We are inlining our function instead of writing a role elsewhere.
On line 2, we take used a bit of ES6 syntax, namely, object destructuring. Whatever data we send via the request gets stored and is available in the trunk
of the req
object. So essentially, we could've replaced line 2 with something similar:
const num1 = req.body.a; const num2 = req.body.b;
On line 3, nosotros are using the transport()
part of the res
object to transport the result of the sum. Again, we are using template literals from ES6. Now to test information technology (using Postman):
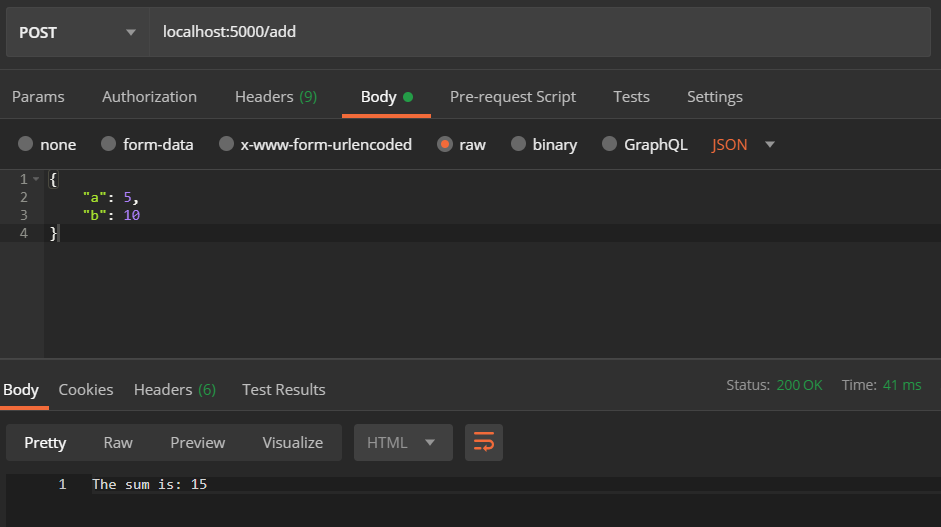
And so we take sent the data 5 and 10 equally a
and b
using them as the torso. Postman attaches this data to the asking and sends it. When the server receives the asking, it can parse the information from req.torso
, as we did in the code above. The issue is shown below.
Alright, the final lawmaking:
const express = require("express"); const bodyParser = require("trunk-parser"); const app = express(); app.apply(bodyParser.json()); const sayHi = (req, res) => { res.transport("Hi!"); }; app.become("/", sayHi); app.post("/add", (req, res) => { const { a, b } = req.body; res.send(`The sum is: ${a + b}`); }); app.heed(5000, () => { panel.log(`Server is running on port 5000.`); });
Residuum Client:
Okay, we have created a server, merely how do we access it from our website or webapp? Here the REST client libraries will come in handy.
We volition exist building a webpage which volition contain a form, where you tin enter two numbers and we will brandish the issue. Let'south beginning.
Beginning, let's change the server.js
a bit:
const path = crave("path"); const express = require("express"); const bodyParser = require("body-parser"); const app = express(); app.use(bodyParser.json()); app.get("/", (req, res) => { res.sendFile(path.join(__dirname, "index.html")); }); app.post("/add", (req, res) => { const { a, b } = req.trunk; res.send({ result: parseInt(a) + parseInt(b) }); }); app.listen(5000, () => { console.log(`Server is running on port 5000.`); });
We imported a new package path
, which is provided by Node, to manipulate path cross-platform. Next we changed the GET
request on '/' and utilise another role available in res
, ie. sendFile
, which allows united states to ship any type of file equally response. Then, whenever a person tries to navigate to '/', they will get our index.html
page.
Finally, we changed our app.mail
function to return the sum as JSON and convert both a
and b
to integers.
Allow'south create an html page, I will phone call it index.html
, with some basic styling:
<!DOCTYPE html> <html lang="en"> <caput> <meta charset="UTF-8" /> <meta proper name="viewport" content="width=device-width, initial-scale=1.0" /> <title>REST Client</title> </head> <style> * { margin: 0; padding: 0; box-sizing: border-box; } .container { height: 100vh; font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, Helvetica, Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol"; brandish: flex; flex-direction: column; justify-content: center; align-items: eye; } form { display: flex; flex-direction: column; margin-bottom: 20px; } label, input[blazon="submit"] { margin-top: 20px; } </style> <body> <div class="container"> <h1>Elementary POST Grade</h1> </h1> <form> <label>Number 1:</label> <input id="num1" type="number" /> <label>Number two:</label> <input id="num2" type="number" /> <input blazon="submit" value="Add"/> </form> <div grade="result">Click Add!</div> </div> </torso> </html>
Permit's add together a script
tag merely before the closing torso tag, so we don't demand to maintain a .js
file. Nosotros will begin by listening for the submit
outcome and call a function accordingly:
<script> document.addEventListener("submit", sendData); </script>
Start we need to prevent page refresh when the 'Add together' push is clicked. This tin be done using the preventDefault()
function. So, we will get the value of the inputs at that instant:
function sendData(eastward) { e.preventDefault(); const a = document.querySelector("#num1").value; const b = document.querySelector("#num2").value; }
Now nosotros will make the call to the server with both these values a
and b
. We will be using the Fetch API, congenital-in to every browser for this.
Fetch takes in 2 inputs, the URL endpoint and a JSON request object and returns a Promise. Explaining them here will be out-of-bounds hither, so I'll leave that for you.
Continue inside the sendData()
function:
fetch("/add", { method: "POST", headers: { Have: "application/json", "Content-Blazon": "awarding/json" }, body: JSON.stringify({ a: parseInt(a), b: parseInt(b) }) }) .then(res => res.json()) .then(data => { const { result } = data; certificate.querySelector( ".event" ).innerText = `The sum is: ${consequence}`; }) .grab(err => console.log(err));
First we are passing the relative URL of the endpoint as the first parameter to fetch
. Next, nosotros are passing an object which contains the method nosotros want Fetch to utilize for the asking, which is POST
in this instance.
We are also passing headers
, which volition provide data near the blazon of information we are sending (content-type
) and the type of data we take as response (accept
).
Next we pass body
. Remember we typed the data equally JSON while using Postman? We're doing kind of a like thing here. Since limited deals with cord as input and processes it according to content-blazon provided, we need to convert our JSON payload into string. We do that with JSON.stringify()
. We're existence a little actress cautious and parsing the input into integers, and so information technology doesn't mess up our server (since we haven't implemented any data-type checking).
Finally, if the promise (returned past fetch) resolves, we will become that response and convert information technology into JSON. After that, nosotros volition go the result from the data
key returned by the response. And so nosotros are simply displaying the result on the screen.
At the stop, if the hope is rejected, nosotros will brandish the error message on the console.
Hither'due south the final lawmaking for index.html
:
<!DOCTYPE html> <html lang="en"> <caput> <meta charset="UTF-8" /> <meta proper name="viewport" content="width=device-width, initial-calibration=1.0" /> <title>Balance Client</title> </head> <style> * { margin: 0; padding: 0; box-sizing: border-box; } .container { meridian: 100vh; font-family: -apple tree-arrangement, BlinkMacSystemFont, "Segoe UI", Roboto, Helvetica, Arial, sans-serif, "Apple tree Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol"; display: flex; flex-direction: column; justify-content: center; marshal-items: centre; } class { display: flex; flex-direction: column; margin-lesser: 20px; } label, input[type="submit"] { margin-top: 20px; } </style> <body> <div course="container"> <h1>Unproblematic POST Form</h1> </h1> <course> <characterization>Number 1:</label> <input id="num1" type="number" /> <label>Number 2:</label> <input id="num2" blazon="number" /> <input type="submit" value="Add"/> </form> <div class="event">Click Add together!</div> </div> <script> document.addEventListener("submit", sendData); function sendData(east) { due east.preventDefault(); const a = document.querySelector("#num1").value; const b = document.querySelector("#num2").value; fetch("/add", { method: "Post", headers: { Accept: "application/json", "Content-Type": "application/json" }, body: JSON.stringify({ a: parseInt(a), b: parseInt(b) }) }) .and so(res => res.json()) .then(data => { const { issue } = data; document.querySelector( ".effect" ).innerText = `The sum is: ${issue}`; }) .catch(err => panel.log(err)); } </script> </body> </html>
I accept spun upwardly a little app on glitch for y'all to test.
Conclusion:
So in this post, nosotros learnt about Remainder architecture and the anatomy of REST requests. We worked our fashion through by creating a simple REST Server that serves Become
and Mail service
requests and built a unproblematic webpage that uses a Residue Client to display the sum of 2 numbers.
You can extend this for the remaining types of requests and fifty-fifty implement a total featured back-end Grime app.
I hope you have learned something from this. If you have any questions, feel complimentary to achieve out to me over twitter! Happy Coding!
Learn to code for gratuitous. freeCodeCamp'southward open up source curriculum has helped more than xl,000 people get jobs equally developers. Get started
Why Should We Prevent A Clientã¢â‚¬â„¢s Direct Access To A Data Structure?,
Source: https://www.freecodecamp.org/news/rest-api-tutorial-rest-client-rest-service-and-api-calls-explained-with-code-examples/
Posted by: eskewbece1940.blogspot.com
0 Response to "Why Should We Prevent A Clientã¢â‚¬â„¢s Direct Access To A Data Structure?"
Post a Comment